Rules for Writing Embedded Software Code: Clean, Maintainable, and Production-Ready
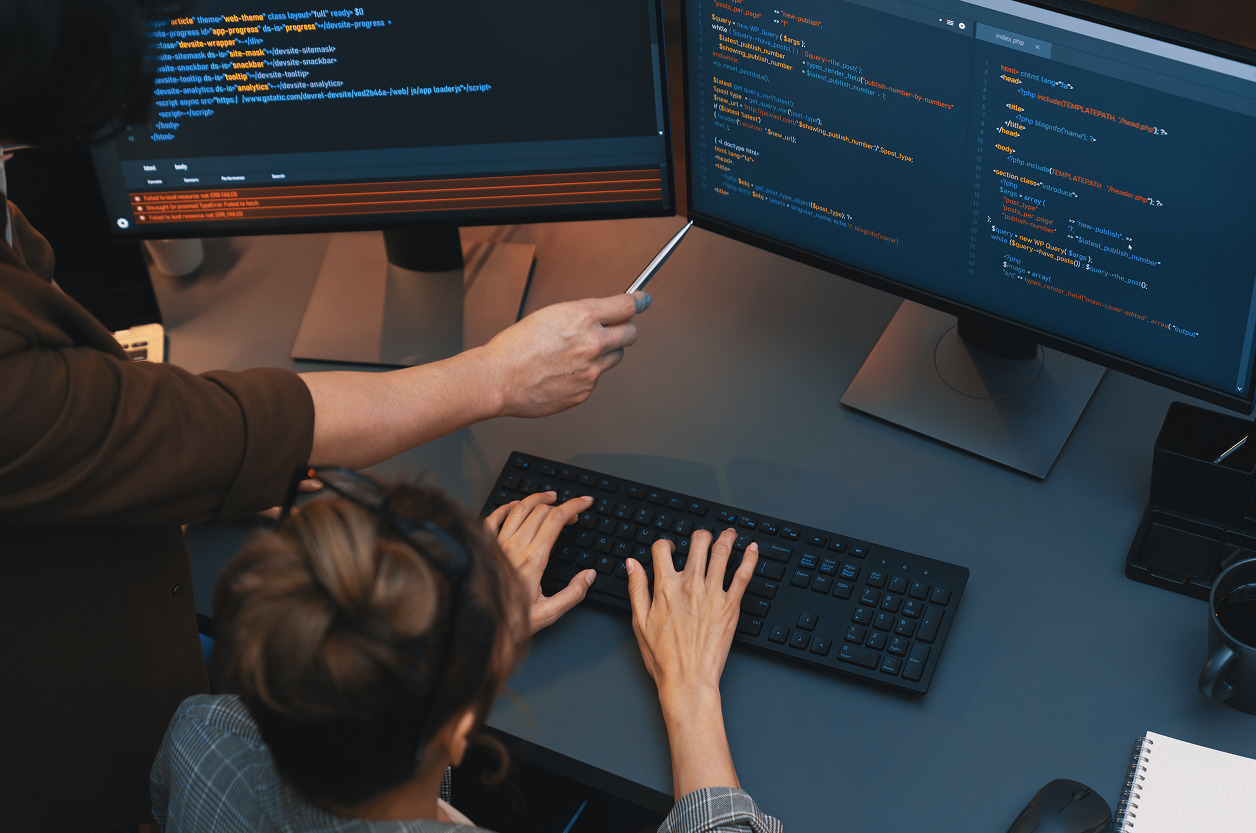
Why Good Code Matters in Embedded Systems
In embedded development, code isn’t just about functionality — it directly impacts system reliability, power consumption, debuggability, and long-term maintainability. Sloppy code can pass initial tests but fail in the field or make future updates nearly impossible.
Whether you're developing bare-metal firmware, RTOS-based drivers, or embedded Linux userland code, adopting proper coding standards helps reduce bugs and enables collaboration.
This article outlines Promwad’s best practices and internal rules for embedded software development across C, C++, and low-level Python when used in tooling.
1. Use Clear and Consistent Naming Conventions
Element | Naming Rule Example |
Functions | void adc_init(void) — lowercase with underscores |
Constants | #define MAX_TEMP_C 85 — all caps + underscores |
Enums | enum adc_channel_t — suffix _t for types |
Structs | typedef struct motor_state_t |
Variables | uint16_t tick_counter — descriptive and typed |
Avoid clever abbreviations. Choose clarity over brevity.
2. Prioritize Modularity and Layering
- Separate hardware abstraction (HAL) from application logic
- Group related functionality in modules (e.g., sensor_temp.c/h)
- Keep ISR handlers minimal — defer processing to task/thread
- Create reusable libraries with clear interfaces
Good modularity makes unit testing and reuse much easier.
3. Be Mindful of Memory and Stack Usage
- Prefer static allocation where possible
- Avoid recursion unless the depth is tightly bounded
- Profile heap/stack usage in production builds
- Use compiler flags for overflow checking (e.g., -fstack-protector)
Embedded devices often run with 32 KB of RAM or less — every byte matters.
4. Follow Consistent Formatting and Style
- 4-space indentation
- Braces on same line for functions and if, for, while
- No mixed tabs/spaces
- Limit lines to 100 characters
- Use clang-format or uncrustify with project config
Clean code is easier to read, debug, and refactor — especially in large teams.
5. Write for Portability
- Avoid compiler-specific extensions unless guarded (#ifdef __GNUC__)
- Use standard types (uint32_t vs unsigned long)
- Abstract platform-dependent parts (e.g., GPIO drivers)
- Avoid hardcoded delays — use timers or RTOS ticks
Portability future-proofs your code for new hardware or RTOS migrations.
6. Validate with Static Analysis and Unit Testing
- Use cppcheck, clang-tidy, or MISRA checkers where applicable
- Set up CI pipelines for automated builds and linting
- Write hardware-independent unit tests with mocks/stubs
- Log coverage metrics for safety-critical modules
Static tools catch what compilers miss — before bugs reach the field.
7. Document the Essentials — Not Everything
Document | What to Include |
Module Header File | Description, function docs, dependencies |
Source File Top | Purpose, revision history, author |
Inline Comments | Non-obvious logic, edge cases |
README (per module) | API examples, config settings |
Avoid over-commenting trivial lines. Aim for clarity through naming.
8. Use Defensive Programming
- Validate all inputs, especially from external interfaces (UART, I2C, etc.)
- Assert preconditions in development builds (assert())
- Check pointer validity and array bounds
- Include timeout logic in loops and state machines
Fail-safe behavior is essential — especially in industrial or safety-critical systems.
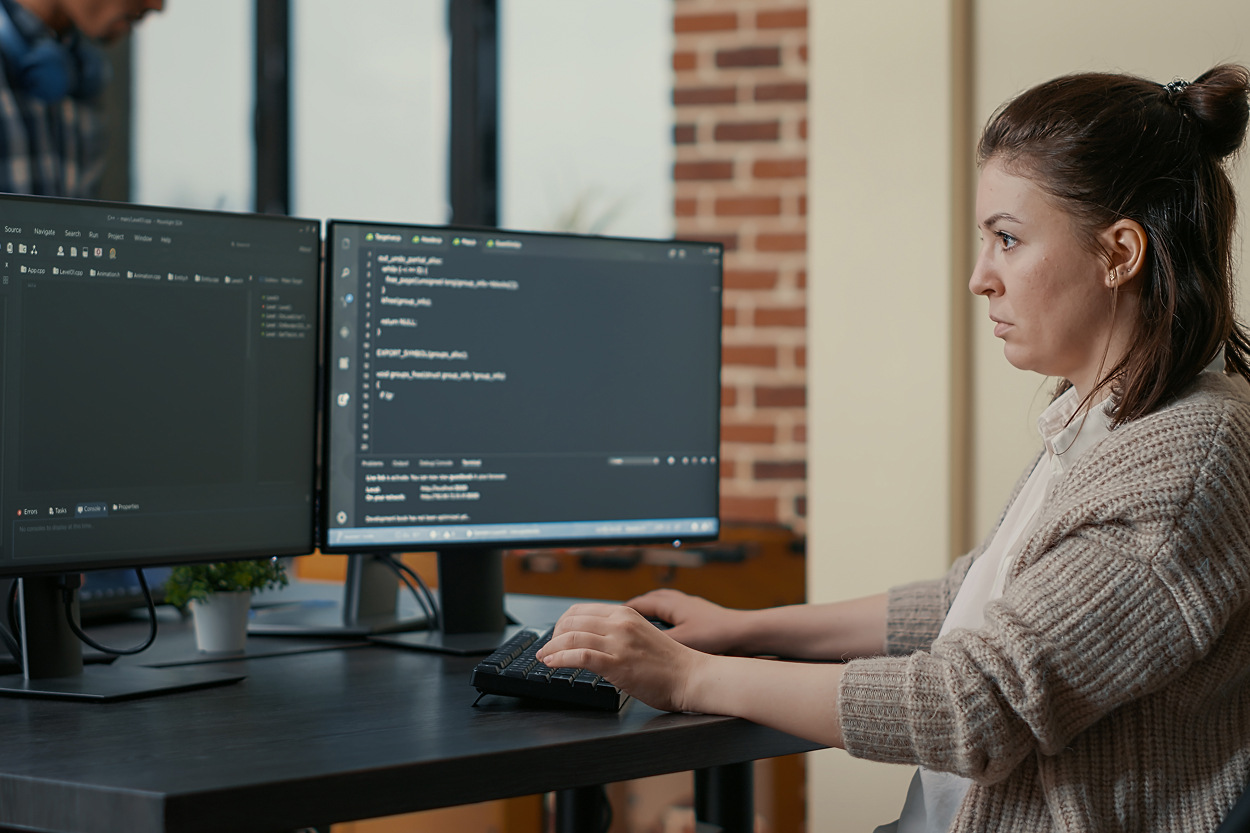
9. Think in State Machines and Event Loops
- Use explicit state enums and transition logic
- Avoid deeply nested if/switch statements
- Model timeouts, retries, and fault handling clearly
- Use frameworks like QPC, libfsm, or write simple internal state handlers
Structured control flow prevents spaghetti code and simplifies debug.
10. Review and Refactor Often
- Code reviews are mandatory before merging
- Refactor regularly — don’t let technical debt accumulate
- Use git blame and commit history to learn from past issues
- Don’t wait until release candidates to clean up — bake quality into the process
Final Thoughts
Embedded software lives close to the hardware — but that doesn’t mean it should be cryptic, fragile, or impossible to maintain.
At Promwad, we treat embedded code as an engineering asset. By following structured rules, we help our clients build safer, leaner, and longer-lasting products.
Let’s write embedded software that scales — not just compiles.
Our Case Studies